Handling dynamic values updated in the controls
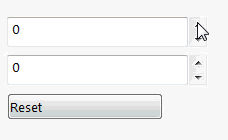
In order to update control values for the properties changed from the code behind dynamically (e.g. on button click or when one property is changing another property), it is required to implement the INotifyPropertyChanged in the data model. Raise the PropertyChanged event for every property which needs to be watched to notify the environment that value has changed and control needs to be updated.
using System; using System.ComponentModel; public class DynamicValuesDataModel : INotifyPropertyChanged { public event PropertyChangedEventHandler PropertyChanged; private double m_Val1; private double m_Val2; public double Val1 { get { return m_Val1; } set { m_Val1 = value; PropertyChanged?.Invoke(this, new PropertyChangedEventArgs(nameof(Val1))); Val2 = value * 2; } } public double Val2 { get { return m_Val2; } set { m_Val2 = value; PropertyChanged?.Invoke(this, new PropertyChangedEventArgs(nameof(Val2))); } } public Action Reset => OnResetClick; private void OnResetClick() { Val1 = 10; } }