Selection box control in SOLIDWORKS Property Page with SwEx.PMPage framework
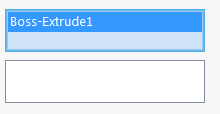
Selection box will be generated for the public properties decorated with SelectionBoxAttribute.
This attribute is applicable to the property of type object or any specific selectable type from SolidWorks.Interop.SldWorks namespace. In this case the type of the object should match the type specified in the SelectionBoxAttribute
public class SelectionBoxDataModel { [SelectionBox(swSelectType_e.swSelSOLIDBODIES)] public IBody2 Body { get; set; } [SelectionBox(swSelectType_e.swSelEDGES, swSelectType_e.swSelNOTES, swSelectType_e.swSelCOORDSYS)] public object Dispatch { get; set; } }
Multiple Selection
This attribute is also applicable to lists. In this case multiple selections will be enabled for the selection box:
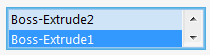
public class SelectionBoxListDataModel { [SelectionBox(swSelectType_e.swSelSOLIDBODIES)] public List<IBody2> Bodies { get; set; } = new List<IBody2>(); [SelectionBox(swSelectType_e.swSelEDGES, swSelectType_e.swSelNOTES, swSelectType_e.swSelCOORDSYS)] public List<object> Dispatches { get; set; } = new List<object>(); }
Additional selection box options can be specified via SelectionBoxOptionsAttribute
Selection Marks
Selection marks are used to differentiate the selection in the selection boxes. In most cases it is required for each selection to come into the specific selection box. In this case it is required to use different selection mark for every selection box. Selection marks are bitmasks, which means that they should be incremented with a power of two (i.e. 1, 2, 4, 8, 16 etc.) in order to be unique. By default SwEx framework will take care of assigning the correct selection marks when this or this version of constructor is used. However it is possible to manually assign the marks using this and this constructors.
Custom selection filters
To provide custom filtering logic for selection box it is required to implement the filter by inheriting the SelectionCustomFilter class and assign the filter via overloaded constructor of SelectionBoxAttribute attribute
public class SelectionBoxCustomSelectionFilterDataModel { public class DataGroup { [SelectionBox(typeof(PlanarFaceFilter), swSelectType_e.swSelFACES)] //setting the standard filter to faces and custom filter to only filter planar faces public IFace2 PlanarFace { get; set; } } public class PlanarFaceFilter : SelectionCustomFilter<IFace2> { protected override bool Filter(IPropertyManagerPageControlEx selBox, IFace2 selection, swSelectType_e selType, ref string itemText) { itemText = "Planar Face"; return selection.IGetSurface().IsPlane(); //validating the selection and only allowing planar face } } }