Get the pointer to component from name using SOLIDWORKS API
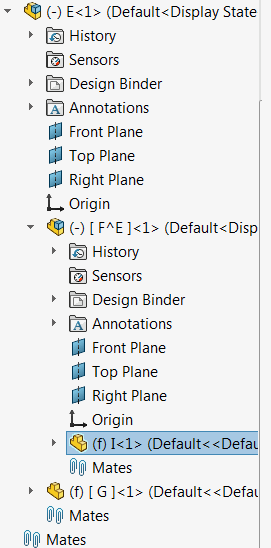
This example demonstrates how to retrieve the pointer to the IComponent2 SOLIDWORKS API method on any level of the assembly from its full name hierarchy.
Name of the component is defined as a path where each level is separated by / symbol. Component instance id is specified with a - symbol (e.g. FirstLevelComp-1/SecondLevelComp-2/TargetComp-1)
Component name can be found in the following dialog in SOLIDWORKS User Interface:
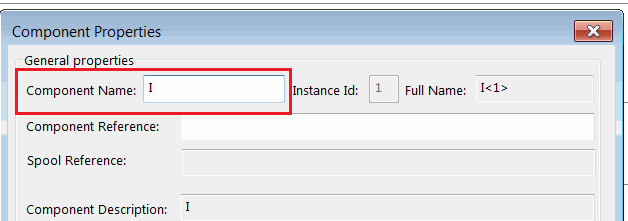
Refer Select Component By Name example for an alternative way of selecting the component by name.
Dim swApp As SldWorks.SldWorks Sub main() Set swApp = Application.SldWorks Dim swAssy As SldWorks.AssemblyDoc Set swAssy = swApp.ActiveDoc Dim swComp As SldWorks.Component2 Set swComp = GetComponentByName(swAssy, "E-1/F^E-1/I-1") If Not swComp Is Nothing Then Debug.Print "Component Found: " & swComp.Name2 swComp.Select4 False, Nothing, False Else Debug.Print "Component Not Found" End If End Sub Function GetComponentByName(assy As SldWorks.AssemblyDoc, name As String) As SldWorks.Component2 Dim vNameParts As Variant vNameParts = Split(name, "/") Dim swComp As SldWorks.Component2 Dim i As Integer For i = 0 To UBound(vNameParts) Dim swCompFeat As SldWorks.Feature If i = 0 Then Set swCompFeat = assy.FeatureByName(vNameParts(i)) Else Set swCompFeat = swComp.FeatureByName(vNameParts(i)) End If If swCompFeat Is Nothing Then Set GetComponentByName = Nothing Exit Function End If Set swComp = swCompFeat.GetSpecificFeature2 Next Set GetComponentByName = swComp End Function