Creating C# add-in for SOLIDWORKS automation using API
- Create new project in Microsoft Visual Studio
- Select Class Library template under the Visual C# templates. Specify the location and the name of the project
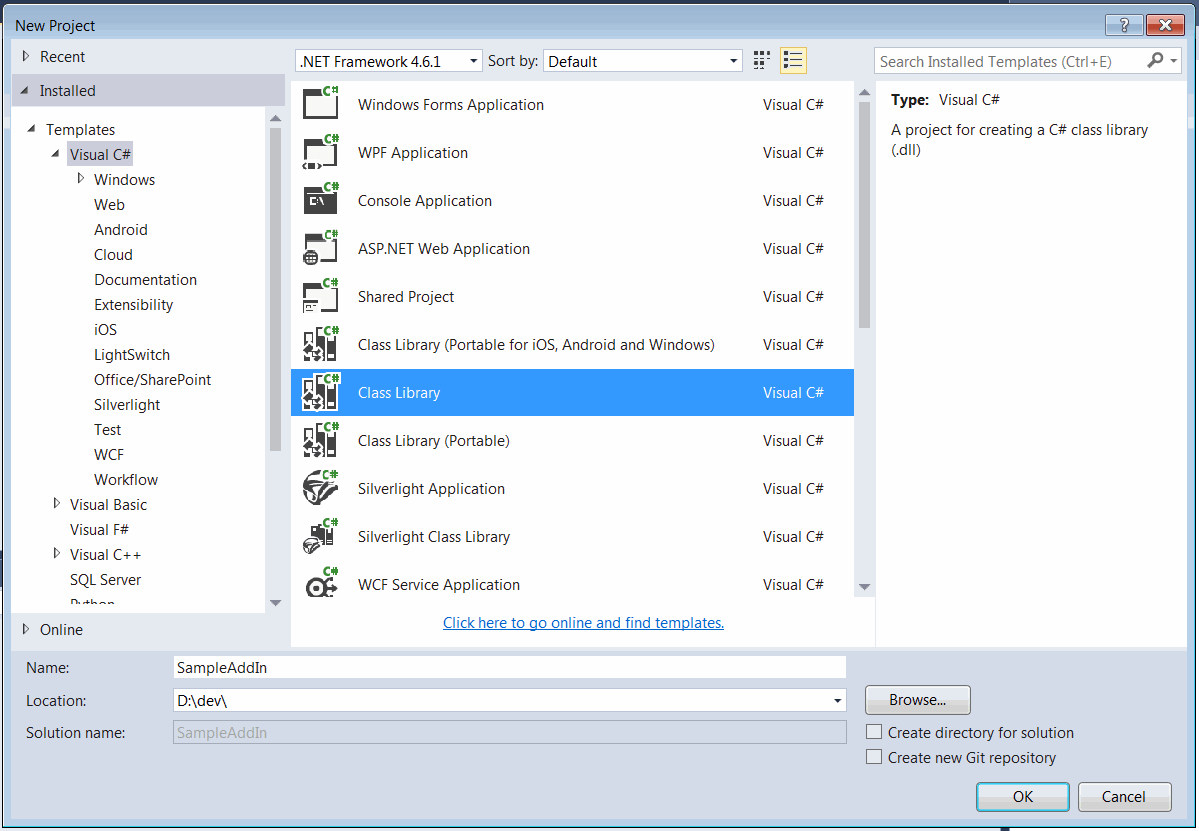
- Add reference to SolidWorks Interop libraries: SolidWorks.Interop.sldworks.dll, SolidWorks.Interop.swconst.dll, SolidWorks.Interop.swpublished.dll. Interop libraries are located at SOLIDWORKS Installation Folder\api\redist for projects targeting Framework 4.0 onwards and SOLIDWORKS Installation Folder\api\redist\CLR2 for projects targeting Framework 2.0 and 3.5.
For projects targeting Framework 4.0 I recommend to set the Embed Interop Types option to false. Otherwise it is possible to have unpredictable behaviour of the application when calling the SOLIDWORKS API due to a type cast issue.
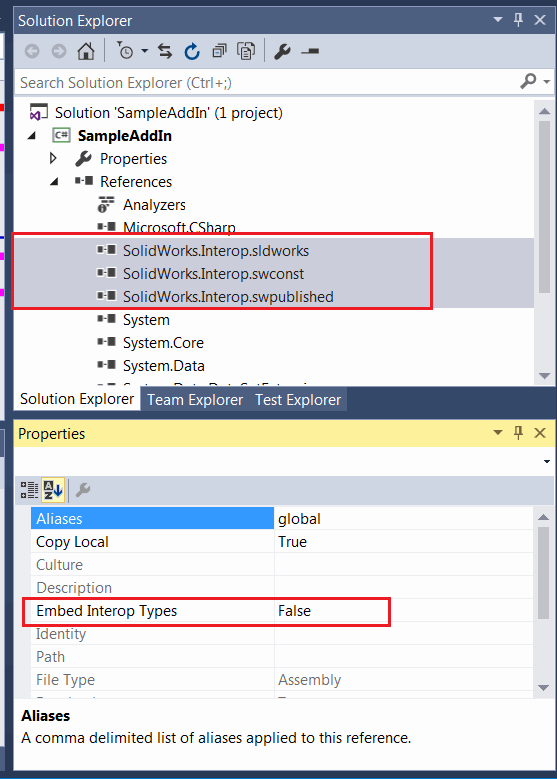
In some tutorials reference to solidworkstools.dll library is added. This library is optional and it won't be used in this tutorial
- Add a public class with a user friendly name. This will be a main class of the add-in. This class must be public and COM-visible. I would recommend to use ComVisibleAttribute to mark the class as COM visible object and GuidAttribute to explicitly assign COM GUID for the add-in class:
[ComVisible(true)] [Guid("31B803E0-7A01-4841-A0DE-895B726625C9")] public class MySampleAddin : ISwAddin { ... }
I would recommend to not select Make assembly COM-Visible option in the project settings but only mark required classes as COM visible as described above.
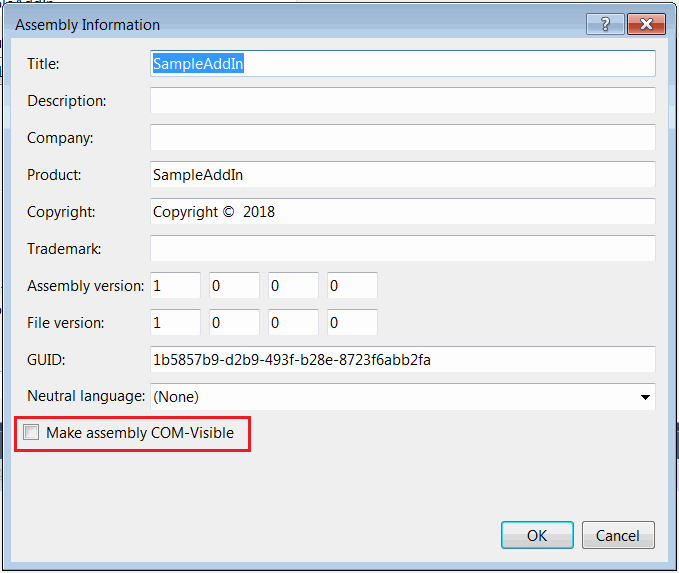
- Add-in dll must be registered with /codebase flag. Register for COM interop options available in the project setting doesn't use this option while registering and not suitable in this case. Instead add the post build action as follows:
"%windir%\Microsoft.NET\Framework64\v4.0.30319\regasm" /codebase "$(TargetPath)"
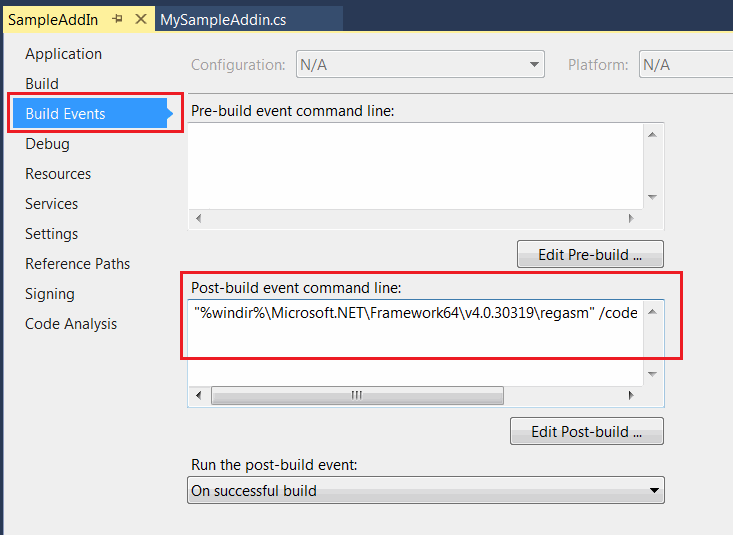
This would ensure the proper registration on each build of the add-in project.
- For the enhanced debugging experience I would recommend to setup the full path to SOLIDWORKS as an external application in project settings.
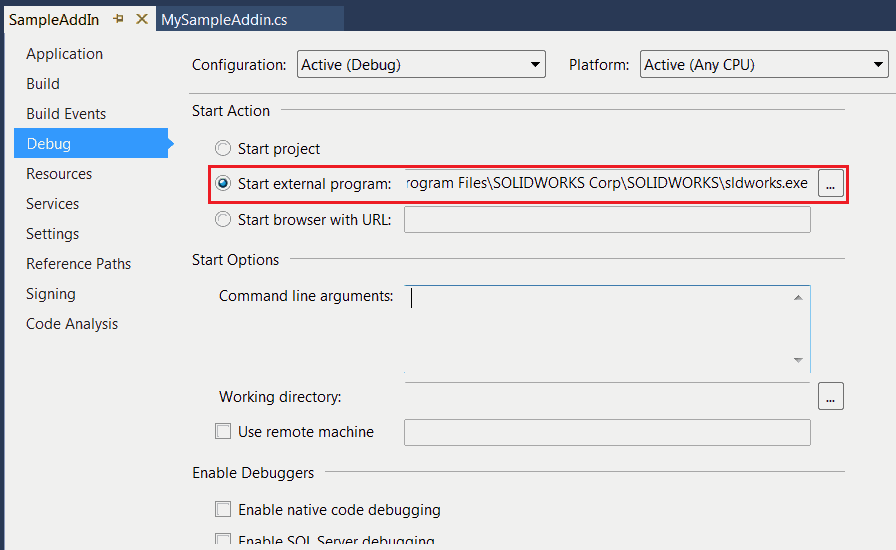
This would allow to start SOLIDWORKS and automatically attach the debugger from the Visual Studio by pressing green run button or F5 key.
Registry information needs to be added to SOLIDWORKS registry branch to make it visible for the application. To simplify the process this information can be automatically added and removed when dll is registered and unregistered as COM object by defining the functions and decorating them with ComRegisterFunctionAttribute and ComUnregisterFunctionAttribute attributes.
Copy paste the code for the add-in as shown below and compile the project
using SolidWorks.Interop.sldworks; using SolidWorks.Interop.swpublished; using System; using System.ComponentModel; using System.Diagnostics; using System.Linq; using System.Runtime.InteropServices; namespace SampleAddIn { [ComVisible(true)] [Guid("31B803E0-7A01-4841-A0DE-895B726625C9")] [DisplayName("Sample Add-In")] [Description("Sample 'Hello World' SOLIDWORKS add-in")] public class MySampleAddin : ISwAddin { #region Registration private const string ADDIN_KEY_TEMPLATE = @"SOFTWARE\SolidWorks\Addins\{{{0}}}"; private const string ADDIN_STARTUP_KEY_TEMPLATE = @"Software\SolidWorks\AddInsStartup\{{{0}}}"; private const string ADD_IN_TITLE_REG_KEY_NAME = "Title"; private const string ADD_IN_DESCRIPTION_REG_KEY_NAME = "Description"; [ComRegisterFunction] public static void RegisterFunction(Type t) { try { var addInTitle = ""; var loadAtStartup = true; var addInDesc = ""; var dispNameAtt = t.GetCustomAttributes(false).OfType<DisplayNameAttribute>().FirstOrDefault(); if (dispNameAtt != null) { addInTitle = dispNameAtt.DisplayName; } else { addInTitle = t.ToString(); } var descAtt = t.GetCustomAttributes(false).OfType<DescriptionAttribute>().FirstOrDefault(); if (descAtt != null) { addInDesc = descAtt.Description; } else { addInDesc = t.ToString(); } var addInkey = Microsoft.Win32.Registry.LocalMachine.CreateSubKey( string.Format(ADDIN_KEY_TEMPLATE, t.GUID)); addInkey.SetValue(null, 0); addInkey.SetValue(ADD_IN_TITLE_REG_KEY_NAME, addInTitle); addInkey.SetValue(ADD_IN_DESCRIPTION_REG_KEY_NAME, addInDesc); var addInStartupkey = Microsoft.Win32.Registry.CurrentUser.CreateSubKey( string.Format(ADDIN_STARTUP_KEY_TEMPLATE, t.GUID)); addInStartupkey.SetValue(null, Convert.ToInt32(loadAtStartup), Microsoft.Win32.RegistryValueKind.DWord); } catch (Exception ex) { Console.WriteLine("Error while registering the addin: " + ex.Message); } } [ComUnregisterFunction] public static void UnregisterFunction(Type t) { try { Microsoft.Win32.Registry.LocalMachine.DeleteSubKey( string.Format(ADDIN_KEY_TEMPLATE, t.GUID)); Microsoft.Win32.Registry.CurrentUser.DeleteSubKey( string.Format(ADDIN_STARTUP_KEY_TEMPLATE, t.GUID)); } catch (Exception e) { Console.WriteLine("Error while unregistering the addin: " + e.Message); } } #endregion private ISldWorks m_App; public bool ConnectToSW(object ThisSW, int Cookie) { m_App = ThisSW as ISldWorks; m_App.SendMsgToUser("Hello World!"); return true; } public bool DisconnectFromSW() { return true; } } }
- When compiled the following warning can be displayed.
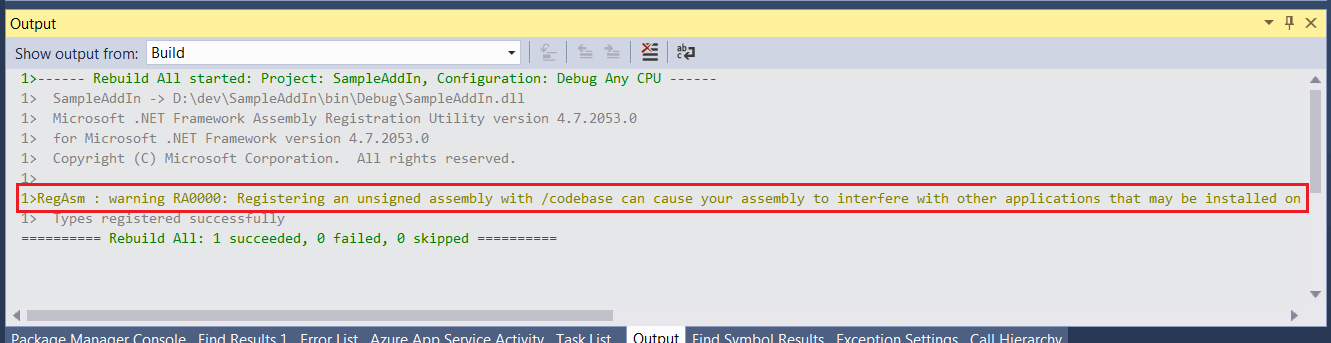
This warning can be ignored.
- Run SOLIDWORKS and the Hello World message box is displayed on start.
The above code can be simplified as shown below with a help of xCAD.NET Framework framework:
[Title("Sample Add-In")] [Description("Sample 'Hello World' SOLIDWORKS add-in")] [ComVisible(true), Guid("31B803E0-7A01-4841-A0DE-895B726625C9")] public class MySampleAddIn : SwAddInEx { public override void OnConnect() { Application.ShowMessageBox("Hello World!"); } }